MULTI THREADING
Multithreading enables programs to perform multiple tasks simultaneously, improving performance and responsiveness
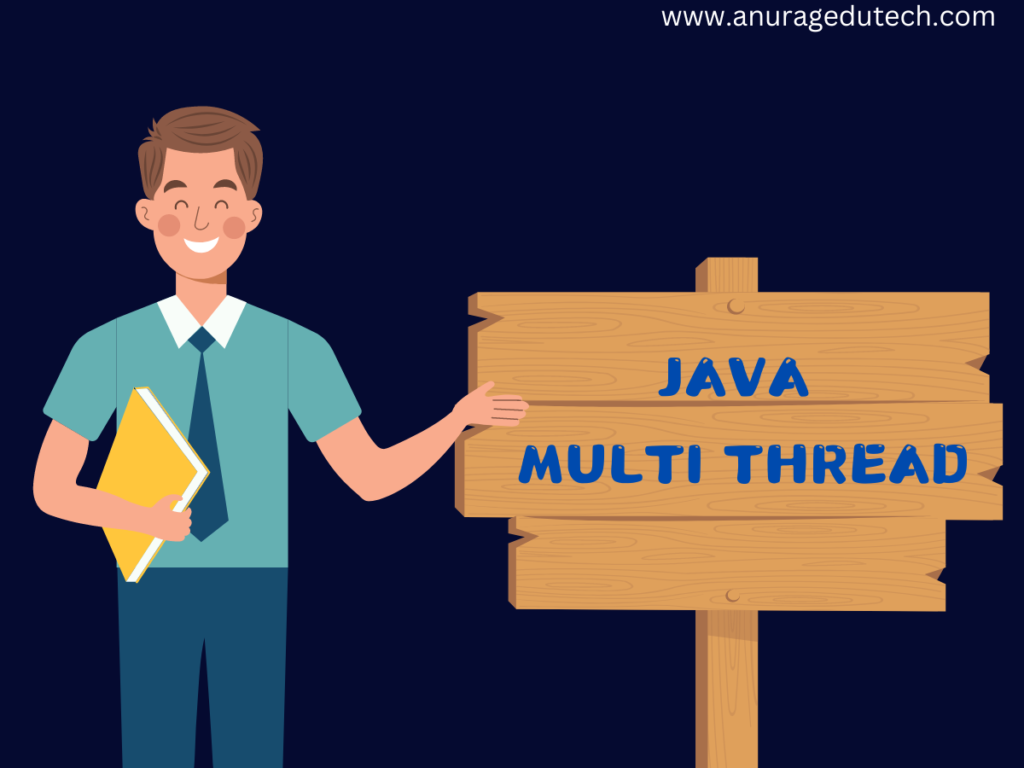
Tread:-
- A flow of control is known as thread.
- If a program contains multiple flows of controls for achieving concurrent execution then that program is known as multi threaded program.
- A program is said to be a multi threaded program If and only if which there exist ‘n’ number of sub-programs there exist a separate flow of control. All such flow of controls are executing concurrently such flow of controls are knows as threads and such type of applications or programs is called multi threaded programs.
- In java program there exist two threads they are fore ground thread and back ground thread.
- Fore ground threads are those which are executing user defined sub-programs where as back ground threads are those which are monitoring the status of fore ground thread.
- There is a possibility of creating ‘n’ number of fore ground threads and always there exist single back ground thread.
- Multi threading is the specialized form of multi tasking of operating system.
Multitasking:-
- Multitasking is a process of executing multiple tasks simultaneously.
- We use multitasking to utilize the CPU Multitasking can be achieved by two ways.
- Process-based multitasking (multiprocessing)
- Thread-based multitasking (multithreading)
Process-based Applications:-
- It is the one in which there exist single flow of control.
- All c, c++ applications comes under it.
- Context switch is more.
- For each and every sub-program there exist separate address pages.
- These are treated as heavy weight components.
- In this we can achieve only sequential execution and they are not recommending for developing internet applications.
Thread-based Applications:-
- It is the one in which there exist multiple flow of controls.
- All java, DOT NET applications comes under it.
- Context switch is very less.
- Irrespective of ‘n’ number of sub-programs there exist single address page.
- These are treated as light weight components.
- In thread based applications we can achieve both sequential and concurrent executions and they are always recommended for developing interact applications.
.
State of thread:
- In JAVA for a thread we have five states. They are
- New state: it is one in which the thread about to enter into main memory.
- Ready state: it is one in which the thread is entered into memory space allocated and it is waiting for CPU for executing.
- Running state: a state is said to be a running state if and only if the thread is under the control of CPU.
- Waiting state: it is one in which the thread is waiting because of the following factors.
- For the repeating CPU burst time of the thread.
- Make the thread to sleep for sleep for some specified amount of time.
- Make the thread to suspend.
- Make the thread to wait for a period of time.
- Make the thread to wait without specifying waiting time.
- Dead state: it is one in which the thread has completed its total execution.
- As long as the thread is in new and halted states whose execution status is false where as when the thread is in ready, running and waiting states that execution states is true.
How to create a Thread:
- By Extending thread Class.
- By implementing Runnable Interface.
Sleeping a thread:
- Public static void sleep(milliseconds);
- Public static void sleep(milliseconds, int nanos ).
Start a thread twice:
- After starting a thread, it can never be started again.
- If you does so, an IllegalThreadStateExceptionis thrown.
- In such case, thread will run once but for second time, it will throw exception.
Calling run() Method:
- Each thread starts in a separate call stack.
- Invoking the run() method from main thread, the run() method goes onto the current call stack rather than at the beginning of a new call stack.
The join() method:
- The join() method waits for a thread to die. In other words, it causes the currently running threads to stop executing until the thread it joins with completes its task.
- Syntax:
public void join()throws InterruptedException public void join(long milliseconds)throws InterruptedException |
getName(),setName(String) and getId() method:
public String getName() |
public void setName(String name) |
public long getId() |
The currentThread() method:
The currentThread() method returns a reference to the currently executing thread object. |
Syntax:
public static Thread currentThread()
Naming Thread:
- The Thread class provides methods to change and get the name of a thread.
- By default, each thread has a name i.e. thread-0, thread-1 and so on.
- By we can change the name of the thread by using setName() method.
The syntax of setName() and getName() methods are given below:
- public String getName(): is used to return the name of a thread.
- public void setName(String name): is used to change the name of a thread.
Priority of a Thread (Thread Priority):
|
3 constants defined in Thread class:
1. public static int MIN_PRIORITY 2. public static int NORM_PRIORITY 3. public static int MAX_PRIORITY |
Default priority of a thread is 5 (NORM_PRIORITY). The value of MIN_PRIORITY is 1 and the value of MAX_PRIORITY is 10. |
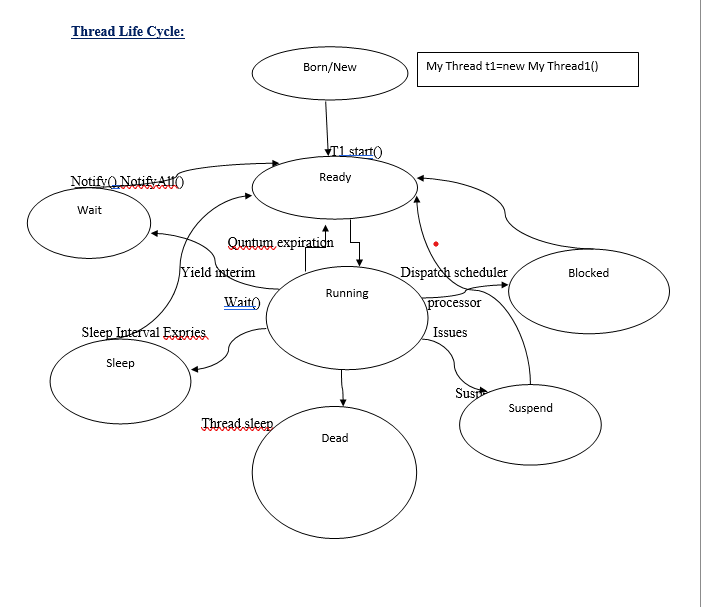